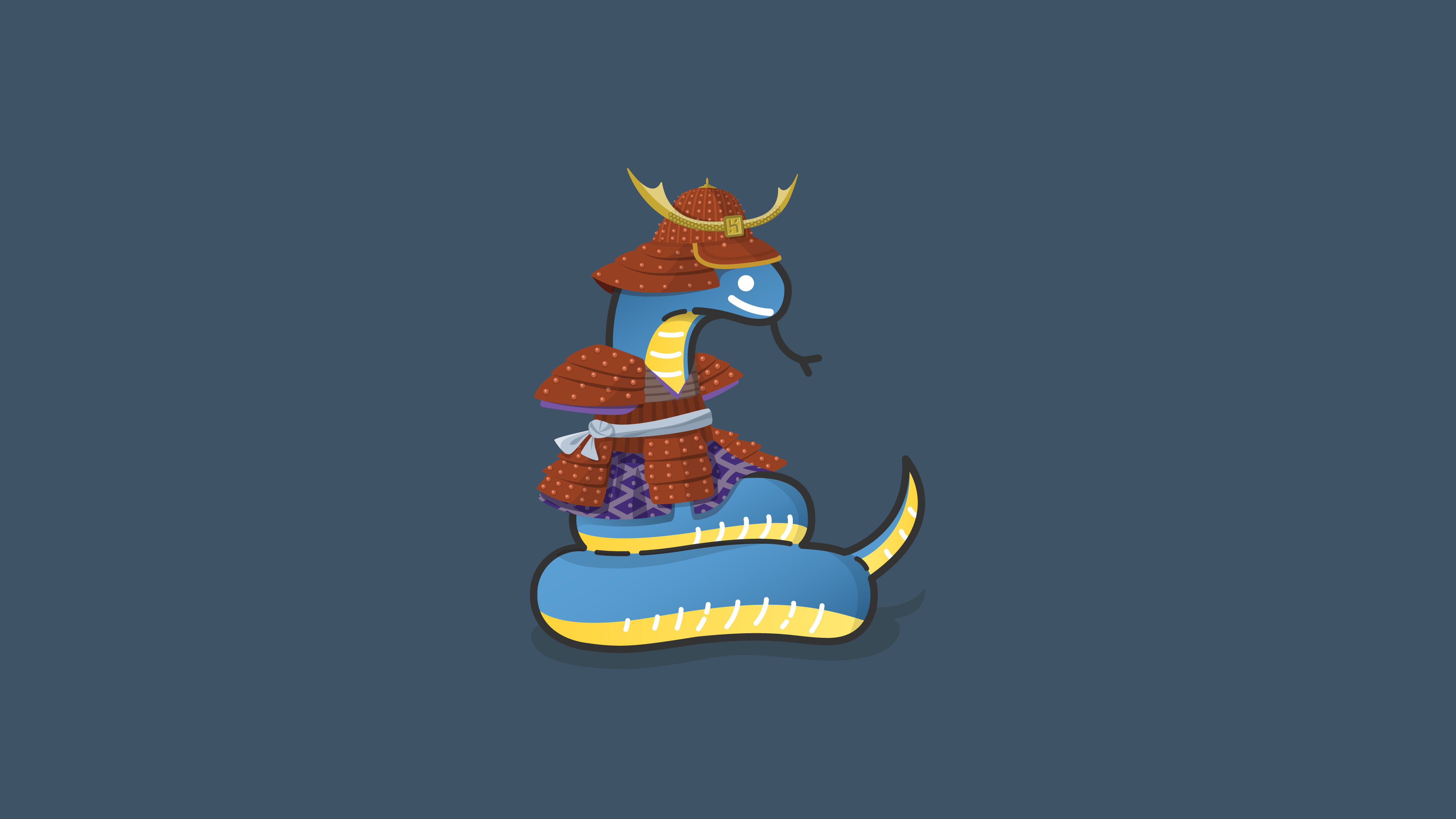
Python: Ultimate Cheat Sheet
๐ Python Basics
Data Structures
Data types
Name | Type | Description | Example |
---|---|---|---|
Integer | int | Whole numbers | -3, 25, 300 |
Floating point | float | Number with a decimal point | -2.3, 4.6, 100.0 |
String | str | Ordered sequence of characters | "hello world!" |
List | list | Ordered sequence of objects | [20, "hello", 4.6] |
Dictionary | dict | Unordered Key:Value pairs | {"name":"Frankie", "surname":"Smith"} |
Tuple | tup | Ordered immutable sequence of objects | [20, "hello", 4.6] |
Set | set | Unordered collection of unique objects | {"a","b","c"} |
Boolean | bool | Binary value | True or False |
Strings
Method | Description | Example | Result |
---|---|---|---|
capitalize() | Convert first character to upper case | ||
count() | Return number of times a specified value occurs in a string | ||
encode() | Returns an encoded version of the string | ||
format() | Format specified values in a string | ||
index() | Return the index position of a specified value | ||
join() | Convert the elements of an iterable into a string | ||
lower() | Convert string into lower case | ||
lstrip() | Trim the left portion of the string | ||
replace() | Replace a specified value with a specified value | ||
rindex() | Return the last position of a specified value | ||
rsplit() | Split the string with a specified seperator and return a list | ||
rsplit() | Trim the right portion of a string | ||
splitlines() | Split the string at line breaks and return a list | ||
strip() | Trim the string | ||
title() | Convert the first character of each word to upper case | ||
upper() | Convert string into upper case |
Lists
Method | Description | Example | Result |
---|---|---|---|
append() | |||
clear() | |||
copy() | |||
count() | |||
extend() | |||
index() | |||
insert() | |||
pop() | |||
remove() | |||
reverse() | |||
sort() |
Dictionaries
Method | Description | Example | Result |
---|---|---|---|
clear() | |||
copy() | |||
fromkeys() | |||
get() | |||
items() | |||
keys() | |||
pop() | |||
popitem() | |||
setdefault() | |||
update() | |||
values() |
Tuples
Method | Description | Example | Result |
---|---|---|---|
count() | Return number of times a specified value occurs | ||
index() | Return index position of a specified value |
Sets
Method | Description | Example | Result |
---|---|---|---|
add() | |||
clear() | |||
copy() | |||
difference() | |||
difference_update() | |||
discard() | |||
intersection() | |||
intersection_update() | |||
isdisjoint() | |||
issubset() | |||
issuperset() | |||
pop() | |||
remove() | |||
remove() | |||
symmetric_difference() | |||
symmetric_difference_update() | |||
union() | |||
update() |
Date and time
Value | Description | Example |
---|---|---|
%a | Abbreviated weekday | Sun |
%A | Weekday | Sunday |
%b | Abbreviated month name | Jan |
%B | Month name | January |
%c | Date and time | |
%d | Day of month | 01 to 31 |
%H | 24 hour | 00 to 23 |
%I | 12 hour | 01 to 12 |
%j | Day of year | 001 to 366 |
%m | Month number | 01 to 12 |
%M | Minute | 00 to 59 |
%p | Time period | AM or PM |
%S | Second | 00 to 61 |
%U | Week number | 00 to 53 |
%w | Weekday | 0 to 6 |
%W | Week number | 00 to 53 |
%x | Date | |
%X | Time | |
%y | Year without century | 00 to 99 |
%Y | Year | 2021 |
%Z | Time zone | GMT |
Operators
Arithmetic operators
Name | Operator | Description | Example | Result |
---|---|---|---|---|
Addition | + | Add a and b | 5+2 | 7 |
Subtraction | - | Subtract b from a | 5-2 | 3 |
Multiplication | * | Multiply a by b | 5*2 | 10 |
Division | / | Divide a by b | 5/2 | 2.5 |
Modulus | % | Remainder of a divided by b | 5%2 | 1 |
Exponent | ** | Raise a to the power of b | 5**2 | 25 |
Integer division | // | Divide a by b and only return integer | 5//2 | 2 |
Comparison operators
Name | Operator | Description | Example | Result |
---|---|---|---|---|
Equal | == | True if a equals b | 5==2 | False |
Not equal | != | True if a is not equal to b | 5!=2 | True |
Less than | < | True if a is less than b | 5<2 | False |
Less than or equal | <= | True if a is less than or equal to b | 5<=2 | False |
Greater than | > | True if a is greater than b | 5>2 | True |
Greater than or equal | >= | True if a is greater than or equal to b | 5>=2 | True |
Logical operators
Operator | Description | Example |
---|---|---|
and | Return True if both statements are true | x>2 and x<5 |
or | Return True if at least one statement is true | x==2 or x>5 |
not | Return False if the statement is true | not(x>2 and x<5) |
Conditional Statements
Statement | Description |
---|---|
if | Execute code if condition is true |
elif | Execute code if previous condition was false |
else | Execute code if all of the previous conditions were false |
pass | Skip executing code of condition is true |
Conditional statement
if a > b:
print("a is greater than b")
elif a == b:
print("a and b are equal")
else:
print("b is greater than a")
Nested if statement
if x > 5:
print("Greater than five")
if x > 10:
print("and greater than ten")
else:
print("and less than ten")
Pass statement
if x > 5:
pass
Loops
For loop
For loop
list = [1,2,3,4,5]
for element in list:
print(element)
# Output: 1,2,3,4,5
Break statement
list = [1,2,3,4,5]
for element in list:
if element == 3:
print("Found!")
break
print(element)
# Output: 1,2,Found!
Continue statement
list = [1,2,3,4,5]
for element in list:
if element == 3:
print("Found!")
continue
print(element)
# Output: 1,2,Found!,4,5
Else statement
list = [1,2,3,4,5]
for element in list:
print(element)
else:
print("Finally finished!")
# Output: 1,2,3,4,5,Finally finished!
Range loop
Range loop
for i in range(10):
print(i)
# Output: 0,1,2,3,4,5,6,7,8,9
Range loop with indexing
for i in range(1,11):
print(i)
# Output: 1,2,3,4,5,6,7,8,9,10
Nested loop
Nested loop
list = [1,2,3]
for element in list:
for letter in "abc":
print(element, letter)
# Output: 1a,1b,1c,2a,2b,2c,3a,3b,3c
While loop
While loop
x = 0
while x < 10:
print(x)
x += 1
# Output: 0,1,2,3,4,5,6,7,8,9,10
Infinite loop with break statement
x = 0
while True:
if x == 5:
break
print(x)
x += 1
# Output: 0,1,2,3,4
Functions
- Function – a collection of instructions that can be initiated by calling a defined function.
- Parameter – the input that you define for the function.
- Argument – value for a given parameter.
- Key word argument – used to make code more readable.
- *args – used for unknown number of arguments. The function will receive a tuple of arguments.
Function
Create a function
def function1():
print("Hi there.")
print("Welcome aboard.")
function1()
# Output: Hi there. Welcome aboard.
Return function
def function2(x):
return 5*x
print(function2(3))
# Output: 15
Multiple argument function
def function3(x, y):
return x+y
print(function3(2,7))
# Output: 9
Function example
def function4(x):
print(x)
print("still in this function.")
return 3*x
print(function4(4))
# Output: 4 still in this function. 12
Function variables
def greet(first_name, last_name):
print(f"Hi {first_name} {last_name}.")
print("Welcome aboard.")
greet("Frankie", "Smith")
# Output: Hi Frankie Smith. Welcome aboard.
BMI calculator example
Input values
name1 = "Frankie"
height1 = 2
weight1 = 90
name2 = "Kelvin"
height2 = 2.5
weight2 = 160
BMI function
def bmi_calculator(name, height, weight):
bmi = weight / (height **2)
print("bmi: ")
print(bmi)
if bmi < 25:
return name + " is not overweight"
else:
return name + " is overweight"
Run function
result1 = bmi_calculator(name1, height1, weight1)
result2 = bmi_calculator(name2, height2, weight2)
# Output:
# bmi: 22.5
# bmi: 25.6
print(result1)
print(result2)
# Output:
# Frankie is not overweight
# Kelvin is overweight
Key-word argument
Key-word argument
def function3(x, y):
return x+y
print(function3(x=2, y=7))
# Output: 9
Arbitrary aruments: *args
*args
# Output:
Arbitrary key-word aruments: **kwargs
**kwargs
# Output:
I’m very happy to uncover this site. I need to to thank you for ones time due to this fantastic read!! I definitely savored every bit of it and i also have you saved to fav to check out new information in your website.